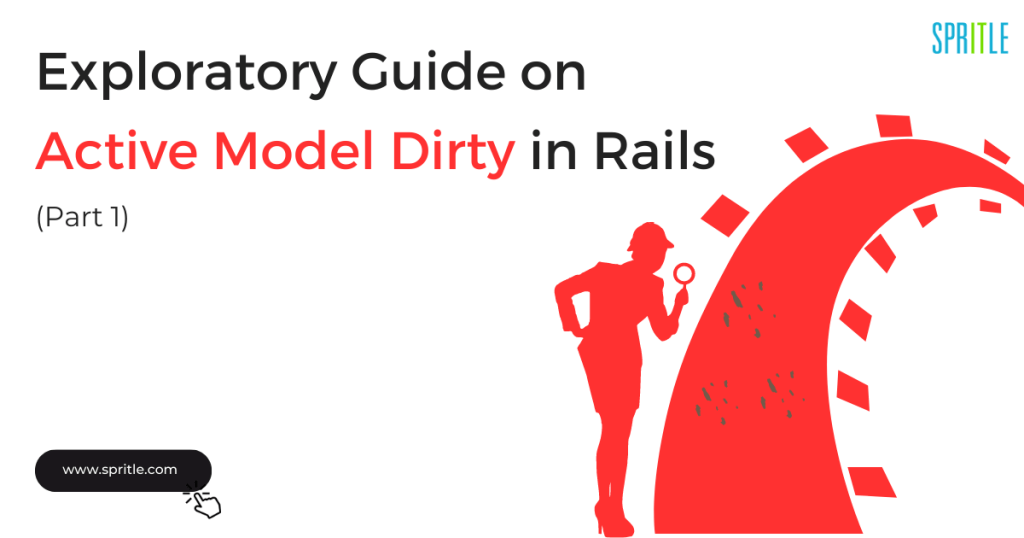
Rails, the superhero of web frameworks, comes packed with amazing features that make our development journey easier. Among its arsenal of superpowers, we have “ActiveModel::Dirty” — a nifty tool that helps us determine if our object attributes have undergone any changes and empowers us to take the appropriate actions accordingly.
Active Model Dirty: Let’s Explore!
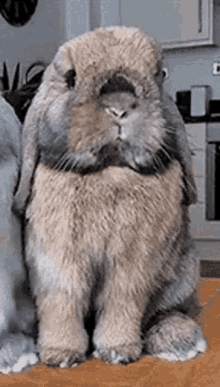
It’s like having a vigilant sidekick keeping an eye on our attribute modifications!
When we modify an attribute on an ActiveRecord object, Rails become our saviour, secretly keeping track of the original attribute value before making the change. This way, we can compare the “before” and “after” values and perform heroic actions based on the changes.
To unlock the power of ActiveModel::Dirty, we simply need to add
include ActiveModel::Dirty
to our object. That’s our secret passphrase to activate the superpowers!
Let’s bring in a model named “Bunny” to the scene:
bunny = Bunny.new
Initially, if we ask our bunny if it has undergone any changes with bunny.changed?
, it might look at us innocently with its floppy ears and say, “Nah, no changes here!” (returning false, of course).
bunny.changed? => false
But fear not, for our bunny is ready for action! Now, let’s assign a value to one of its attributes, such as the “name” attribute:
bunny.name = 'Vargheese'
In a flash, if we call the Dirty methods:
bunny.changed? => true
bunny.name_changed? => true
bunny.name_was => nil
bunny.name_change => [nil, "Vargheese"]
See? Our bunny is now proudly proclaiming, “I’ve been changed! Look, my name was nil, but now it’s ‘Vargheese’! Witness my transformation!”
But wait, there’s more! If we try to be mischievous and assign the same value “Vargheese” again, our bunny will play it cool and say, “Nice try, but that doesn’t count as a change. I’m onto your tricks!”
These cunning Dirty methods only track unsaved changes. Once we save our bunny with a triumphant bunny.save!
, it becomes calm and composed, assuring us that it has no pending changes with bunny.changed?
returning false.
But what if we want to take a peek at the changes even after saving? Fear not, for our bunny has a secret stash of information! With bunny.previous_changes
, it spills the beans and reveals the history of modifications. We can now examine all the attributes that underwent changes, including timestamps like “created_at” and “updated_at”.
bunny.previous_changes => {"name" => [nil, "Vargheese"], "created_at" => [nil, Sun, 02 Jul 2023 09:30:34.553698000 UTC +00:00], "updated_at" => [nil, Sun, 02 Jul 2023 09:30:34.553698000 UTC +00:00] }
bunny.name_previously_changed? => true
bunny.name_previous_change => [nil, "Vargheese"]
Now, our bunny turns into a storyteller, regaling us with tales of its past transformations and the timestamps that witnessed its evolution!
In case we want to start afresh, our bunny can hit the “reload” button and wipe the slate clean:
bunny.reload
bunny.previous_changes => {}
bunny.name => "Vargheese"
It’s like our bunny has found a time machine and reset itself to its original state, ready to embark on new adventures!
Oh, and did I mention our bunny has a restore_attributes
superpower too? If we make further changes like bunny.name = 'Carlos'
, it can simply use its power with bunny.restore_attributes
and revert back to the original values, saying, “Sorry, Carlos, but I’m sticking with my original name!”.
bunny.name = "Carlos"
bunny.restore_attributes => ["name"]
bunny.name => "Vargheese"
And there you have it! ActiveModel::Dirty tracking in Rails is like having a loyal sidekick by your side, helping you track and respond to changes in your application’s data.
Now, we have covered most of the methods in Active Model Dirty like
- [attr_name]_changed?
- [attr_name]_change
- reload
- [attr_name]_previously_changed?
- [attr_name]_was
Stay Tuned for The Next Post!
So, in the next post, let’s wonder how we can use Bunny’s super power (ActiveModel:: Dirty methods) in real-time application scenarios and use it in full working real-time functionality with more Dirty methods!