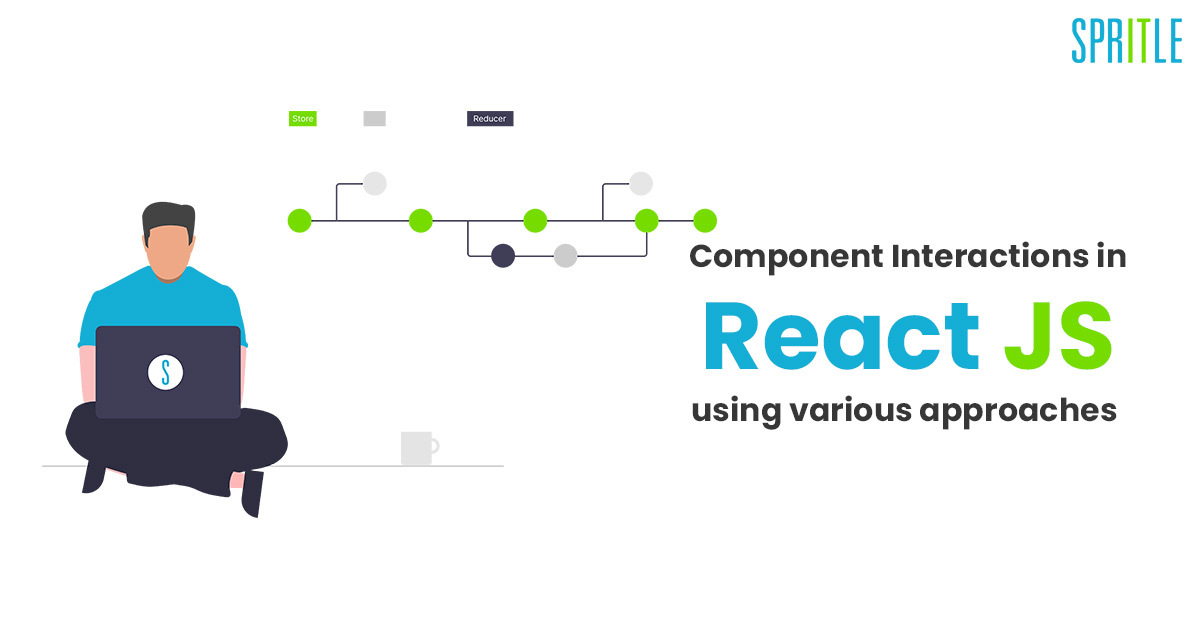
In any Single Page Application (SPA) that is built using JavaScript libraries or frameworks, there’s a need for the components to communicate with the data either from parent to child or from a child to parent components.
Let us now discuss the possibilities of handling the component interactions, a key aspect of building applications in React.
Understanding Props and Callbacks:
Component interactions happen both ways between parent and child. To handle the to-and-fro movements, we break the interaction strategies into concepts like Props and Callbacks.
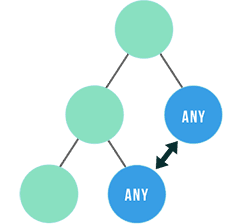
Assuming the project scaffolding in the below hierarchy,
App
└── ParentComponent1
├── ChildComponent1
└── ChildComponent2
└── ParentComponent2
Props – from parent to child:
Passing data from parent to child or to a Higher-Order Component (HOC) can be achieved using the props. For example, passing a name property into a child component from a parent can be done using the below snippet.
const ParentComponent1 = () => <ChildComponent1 title="Component Interactions in React" />
const ChildComponent1 = (props) => <h2> Blog Title: <span>{props.title}</span></h2>
Callbacks – from child to parent:
When a parent needs to trigger a method from a child component for some scenarios where the button placed in the parent component needs to access a method inside a child component. There are multiple ways to achieve this.
Callback as a function:
Here, a parent-child combination is taken as an example to show how the callbacks can be used to update the title of a blog from the child component ChildComponent2
to its parent component ParentComponent1
.
const ParentComponent1 = () => {
const [title, setTitle] = React.useState('');
return (
<h2> Blog Title: <span>{title}</span></h2>
<ChildComponent2 updateParentCallback={(updatedTitle) => setTitle(updatedTitle) />
)
}
const ChildComponent2 = (props) => {
const updateTitle = (updatedTitle) => {
props.updateParentCallback(updatedTitle)
}
return (
<h4>This is child component 2</h2>
<button onClick={() => updateTitle('Component Interaction for callbacks')>Update Title</button>
)
}
Instance methods – UseRef:
Instance methods on the child component can be called by the parent using refs. This is another way to communicate with a child from a parent.
Just three steps are required to set up the instance methods:
- Define a method in the child component
- Add a ref attribute that creates a reference to the child component
ChildComponent2
in the parent componentParentComponent1
. - Call the child component method from the parent.
const ParentComponent1 = () => {
const [title, setTitle] = React.useState('');
const updateTitle = (updatedTitle) => {
const newTitle = childRefComponent.updateTitle(updatedTitle);
setTitle(newTitle);
}
return (
<h2> Blog Title: <span>{title}</span></h2>
<button onClick={() => updateTitle('Component Interaction for callbacks')>Update Title</button>
<ChildComponent2 ref={childRef => childRefComponent = childRef} />
)
}
const ChildComponent2 = (props) => {
const updateTitle = (updatedTitle) => {
return updatedTitle + ' - suffix defined in child component';
}
return (
<h4>This is child component 2</h2>
)
}
Instance methods – UseImperativeHandle:
useImperativeHandle
customizes the instance value that is exposed to parent components when using ref
. As always, imperative code using refs should be avoided in most cases.
useImperativeHandle
should be used with forwardRef
const ParentComponent1 = () => {
const childRef = React.useRef(null);
const [title, setTitle] = React.useState('');
const updateTitle = (updatedTitle) => {
const newTitle = childRef.current.updateTitle(updatedTitle);
setTitle(newTitle)
}
return (
<h2> Blog Title: <span>{title}</span></h2>
<button onClick={() => updateTitle('Component Interaction for callbacks')>Update Title</button>
<ChildComponent2 ref={childRef} />
)
}
const ChildComponent2 = React.forwardRef((props, ref) => {
React.useImperativeHandle(forwardedRef, ()=>({
updateTitle() {
return updatedTitle + ' - suffix defined in child component';
}
});
return (
<h4>This is child component 2</h2>
)
})
Redux:
Redux is an efficient way of passing props into components irrespective of their hierarchy. It’s basically a single state tree maintained outside the component hierarchy but which is designed to connect easily to your components, allowing sideways access to data.
Below is a simple animation to understand the flow and how it works.
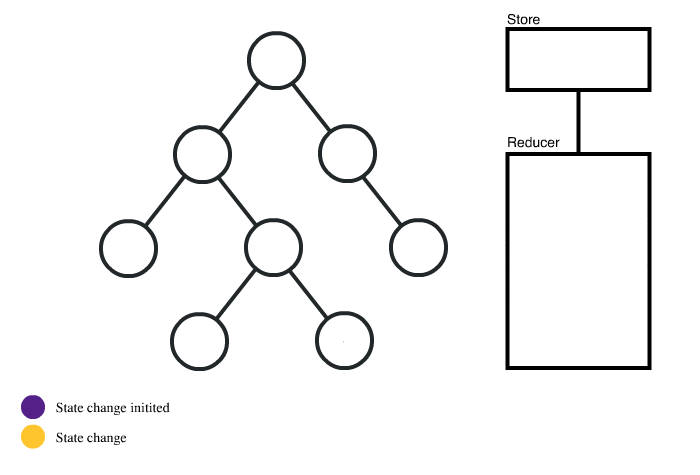
React Context API:
React Context API works pretty similar to Redux without importing external plugins like react-redux
. But, this is available internally with React. Let’s try and implement this to see how it works.
Firstly, we need to add a provider at the top level of the application.
const App = () => {
const ContextProvider = React.createContext();
return (
<ContextProvider>
<ParentComponent1 />
<ParentComponent2 />
</ContextProvider>
}
}
And wherever the data needs to be passed, we can access it using useContext()
. Let’s say we need to access the data into a child component ChildComponent2
. And here’s how we can do it.
const ChildComponent - () => {
const store = React.useContext(TitleContext);
return (
<h2> Blog Title: <span>{store.title}</span></h2>
)
}
These are a few of the methods that I follow to handle the data interactions among components. But, It’s totally up to a Developer to decide on which one to use based on the scale of the project, making it more efficient.
Hope this blog gives you an idea of how to handle data interactions. I have done it my way. You have figured out your own way? Then share it in the comments section below. And also, do not forget to reach out for any discussions.
Happy Coding!
Awesome Abu